MapLibre Native for Android serves as a toolkit, empowering developers to easily integrate dynamic map displays with scalable, adaptable vector maps of Galli Maps into their Android applications.
In this guide, we'll walk you through the integration of Maplibre Native into your Android Kotlin application with step-by-step examples.
To get started, you'll need a Galli Maps access token. Simply SIGN UP to create
an account and generate access token to start implementing our services.
1. Integrating GalliMaps with Maplibre
#### Step 1: Add Maplibre Dependency
Add Maplibre Native dependency to your `build.gradle` file.
dependencies {
implementation 'org.maplibre.gl:android-sdk:10.0.2'
. . .
. . .
}
#### Step 2: Set Up MapView in XML
Define `MapView` in your layout XML file.
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<com.mapbox.mapboxsdk.maps.MapView
android:id="@+id/mapView"
android:layout_width="match_parent"
android:layout_height="match_parent"
/>
</androidx.constraintlayout.widget.ConstraintLayout>
#### Step 3: Initialize MapView and Load Galli Maps’ Vector Tile
In the activity page, initialize MapLibre's MapView and load Galli Maps' vector tiles URL. To load the map tiles, you will need to include an access token in the tile URL to authenticate and render the tiles onto the MapView.
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.view.LayoutInflater
import com.mapbox.mapboxsdk.Mapbox
import com.mapbox.mapboxsdk.camera.CameraPosition
import com.mapbox.mapboxsdk.geometry.LatLng
import com.mapbox.mapboxsdk.maps.MapView
class MainActivity : AppCompatActivity() {
private lateinit var mapView: MapView
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
// Replace ACCESS-TOKEN with your access token
val galliTileUrl= "https://map-init.gallimap.com/styles/light/style.json?accessToken=[ACCESS-TOKEN]"
// Init MapLibre
Mapbox.getInstance(this)
// Init layout view
val inflater = LayoutInflater.from(this)
val rootView = inflater.inflate(R.layout.activity_main, null)
setContentView(rootView)
// Init the MapView
mapView = rootView.findViewById(R.id.mapView)
mapView.getMapAsync { map ->
map.setStyle(galliTileUrl)
map.cameraPosition = CameraPosition.Builder().target(LatLng(27.69818, 85.32385)).zoom(12.0).build()
}
}
override fun onStart() {
super.onStart()
mapView.onStart()
}
override fun onResume() {
super.onResume()
mapView.onResume()
}
override fun onPause() {
super.onPause()
mapView.onPause()
}
override fun onStop() {
super.onStop()
mapView.onStop()
}
override fun onLowMemory() {
super.onLowMemory()
mapView.onLowMemory()
}
override fun onDestroy() {
super.onDestroy()
mapView.onDestroy()
}
override fun onSaveInstanceState(outState: Bundle) {
super.onSaveInstanceState(outState)
mapView.onSaveInstanceState(outState)
}
}
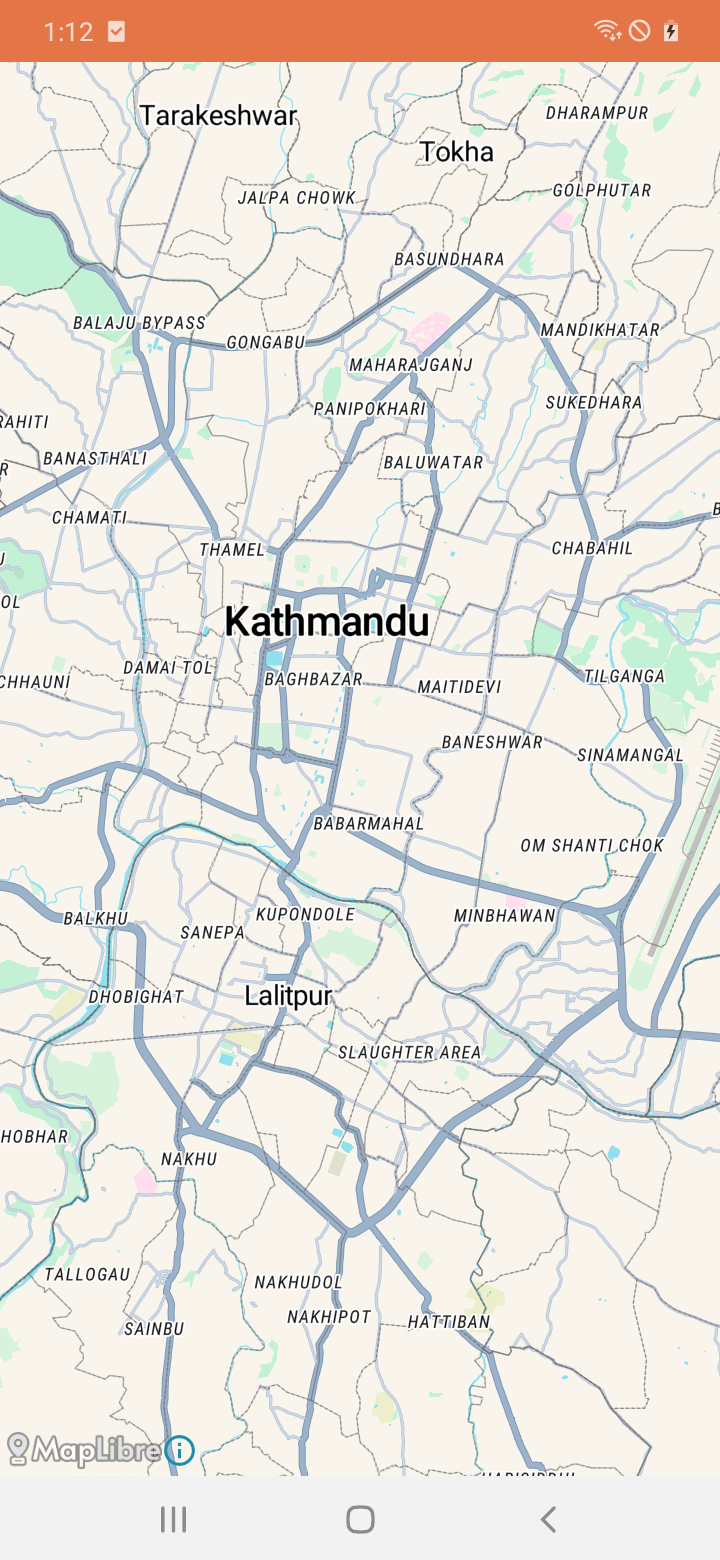
Loading Galli Maps' Vector Tiles
2. Pin Marker on Map [ Example ]
This example shows how to add a marker (pin) on the map at a specific geographical position defined by latitude and longitude. It creates a marker with options such as position and title, then adds it to the map.
mapView.getMapAsync { map ->
...
...
val options = MarkerOptions()
.position(LatLng(27.69818, 85.32385))
.title("Marker Title")
map.addMarker(options)
...
...
}
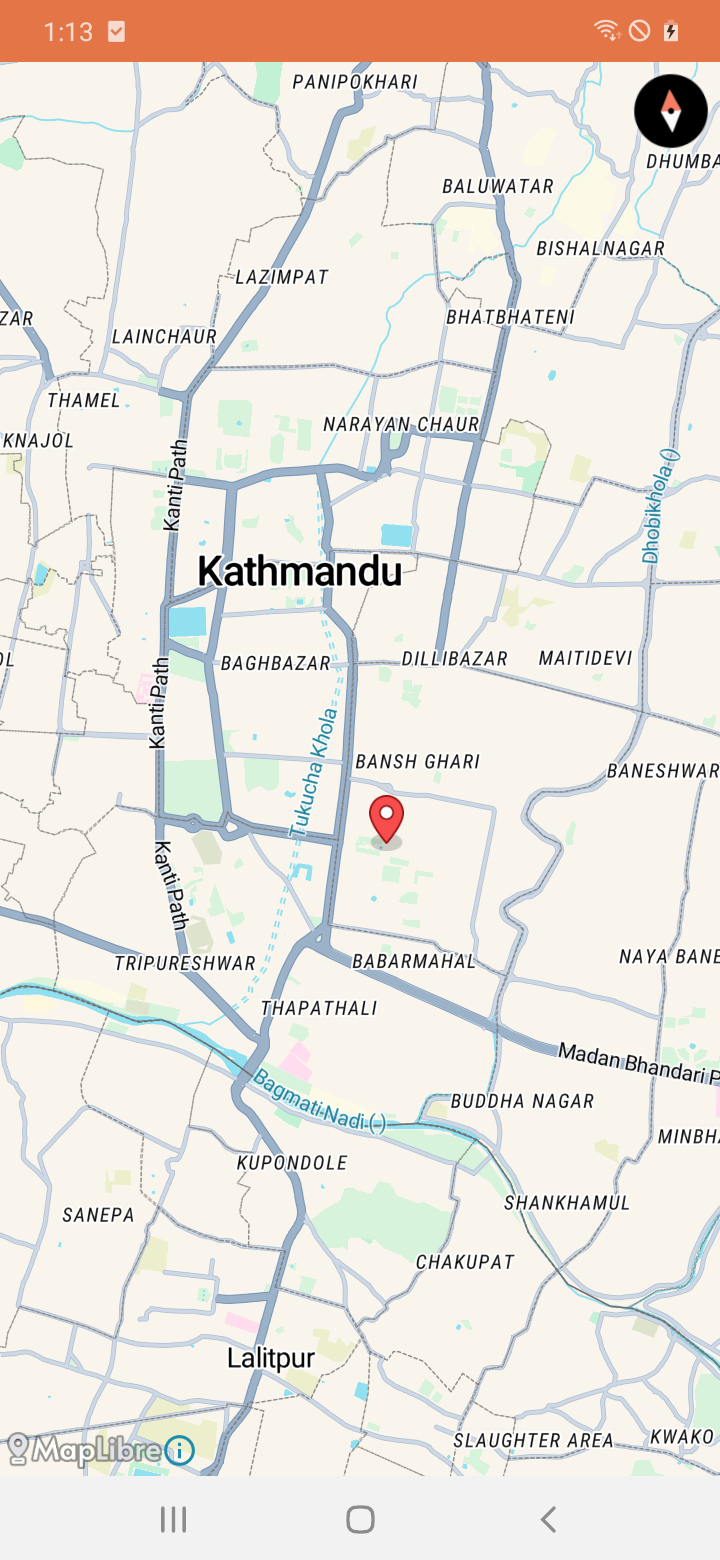
Pin marker on map
3. Draw a Polyline on Map [ Example ]
Here, we draw a polyline on the map by connecting multiple geographical points. It creates a polyline with options such as color and width, then adds it to the map.
mapView.getMapAsync { map ->
...
...
val polylineOptions = PolylineOptions().add(LatLng(27.69818, 85.32385), LatLng(27.66918, 85.32034))
.color(Color.RED)
.width(2f)
map.addPolyline(polylineOptions)
...
...
}
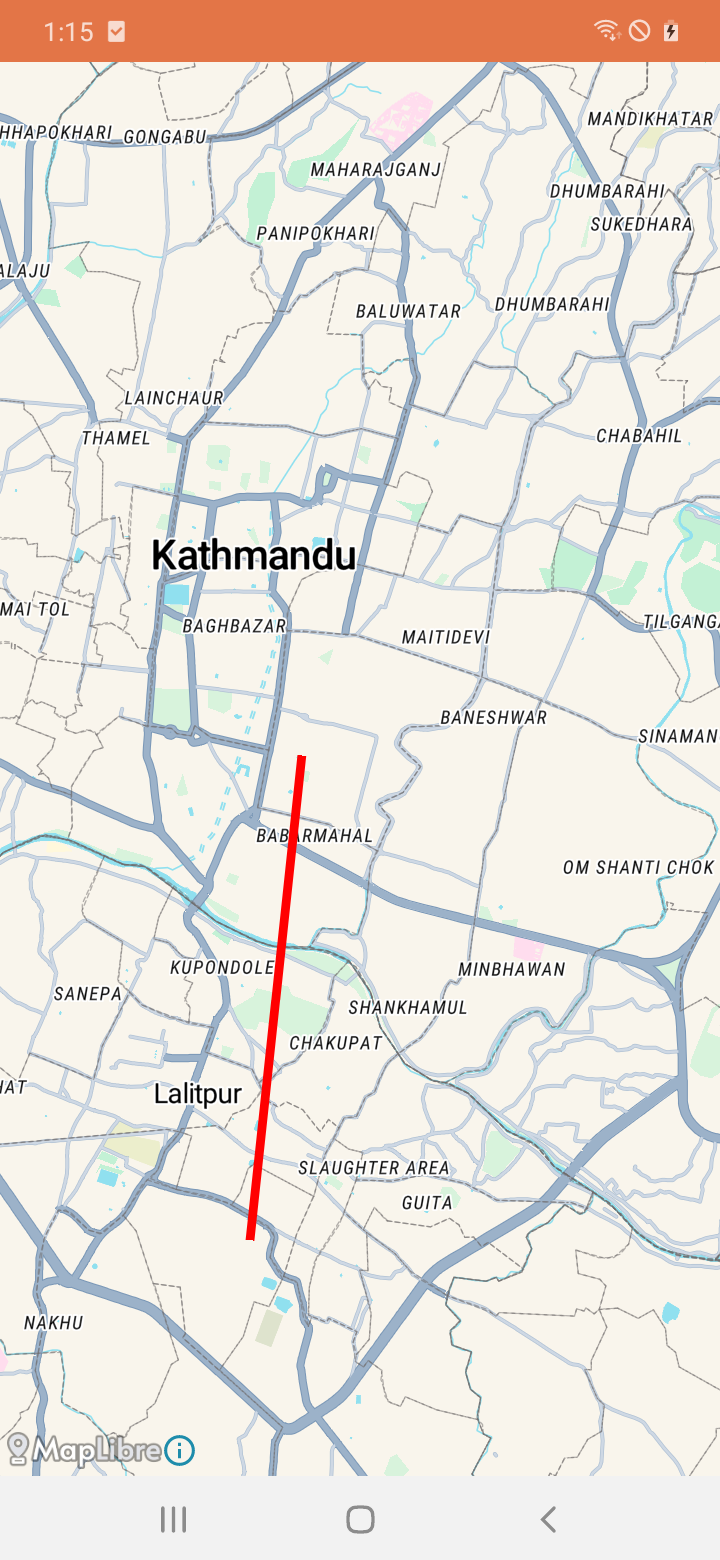
Polyline on map
4. Draw a Polygon on Map [ Example ]
Similar to drawing a polyline, this example demonstrates drawing a polygon on the map by defining its vertices (points). It creates a polygon with options such as fill and stroke colors, then adds it to the map.
mapView.getMapAsync { map ->
...
...
val polygonOptions = PolygonOptions()
.addAll(listOf(LatLng(27.72441,85.29000), LatLng(27.72206, 85.34864), LatLng(27.67013, 85.34181)))
.fillColor(Color.parseColor("#3bb2d0"))
.strokeColor(Color.parseColor("#ffffff"))
.strokeWidth(2f)
map.addPolygon(polygonOptions)
...
...
}
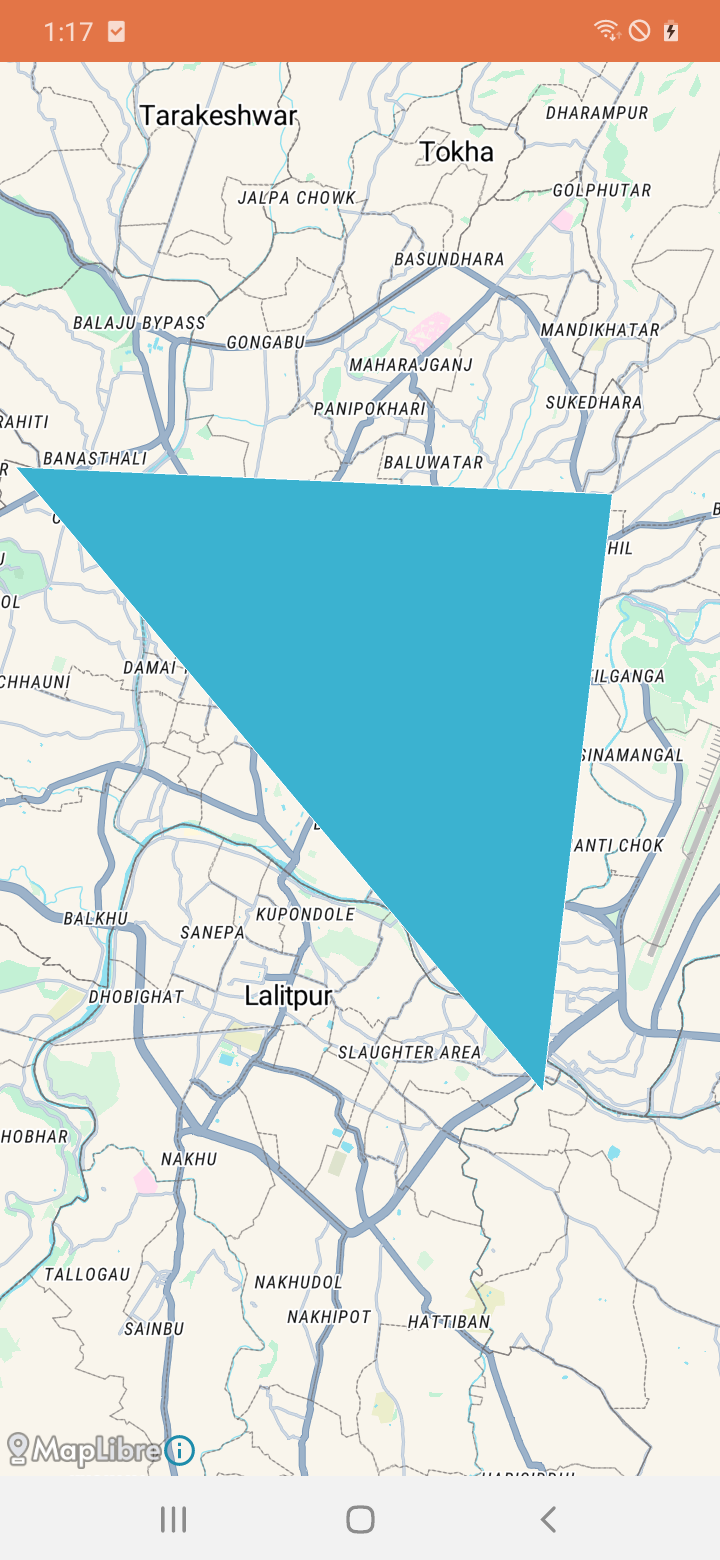
Polygon on map
5. Execute an Action on Map Tap [ Example ]
In this example, we handle map tap events. Whenever a user taps on the map, the specified action will be executed. The `addOnMapClickListener` method adds a listener to the map, which triggers when the map is tapped, allowing you to perform custom actions in response to the tap event.
mapView.getMapAsync { map ->
...
...
map.addOnMapClickListener { point ->
// Handle map tap event
val options = MarkerOptions()
.position(point)
.title("Marker Title")
map.addMarker(options)
true
}
...
...
}
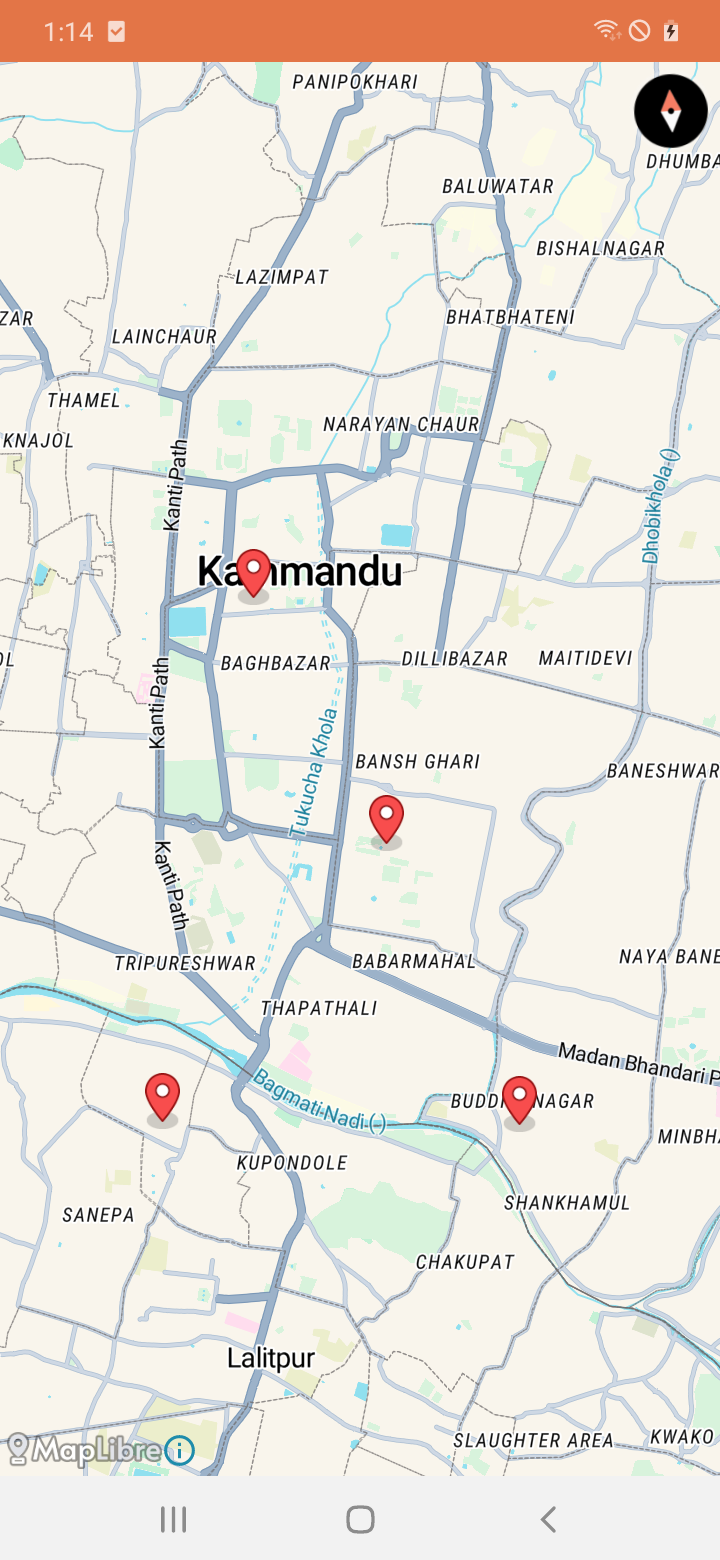
On Tap function on map
For more information on maplibre sdk for android please refer to following page: Maplibre Native (Android)