The GalliMap Plugin is a JavaScript library that enables the integration of GalliMaps into your web application.
Include GalliMaps CSS
<link rel="stylesheet" href="https://gallimap.com/static/dist/css/gallimaps.min.css">
Include GalliMaps JS
<script src="https://gallimap.com/static/dist/js/gallimaps.min.js"></script>
DEMO WEBSITE
Display Map
This section shows how to embed GalliMaps map into website using [gallimap_plugin.js] and [gallimap.css].
<!DOCTYPE html>
<html lang="en">
<head>
<title>Galli Maps</title>
<meta charset="utf-8" />
<meta http-equiv='X-UA-Compatible' content='IE=edge'>
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="shortcut icon" type="image/x-icon" href="docs/images/favicon.ico" />
<link rel="stylesheet" href="[gallimap.css]">
</head>
<body>
<div id="map"></div>
<script src="[gallimaps_plugin.js]"></script>
<script>
gallimapsConfig.accessToken="[ACCESS-TOKEN]";
let map = displayMap(19, 13, [27.6772138, 85.3222383]);
</script>
</body>
</html>
Set [ACCESS-TOKEN]
gallimapsConfig.accessToken=[ACESS-TOKEN]
gallimapsConfig.accessToken | Required for accessing the Map and extra resource which will be provided after registering in GalliMaps |
SCRIPT:
let map = displayMap(maxZoom, minZoom, latlng);
maxZoom | Maximum zoom level of map. Maximum value is 18. Value should be non-negative. |
minZoom | Minimum zoom level of map. Minimum value is 13. Value should be non-negative. |
latlng | Set the center coordinates of the map [latitude,longitude] |
Galli 360
This section demonstrates how to display 360 image markers in the map and display 360 panoroma image in viewer
HTML
<div class="image-container" id="pano"> </div>
SCRIPT:
let pinMap = { iconUrl : '', iconSize : [20,20], iconAnchor : [20,20] };
let mapClicked = { iconUrl : './images/marker-icon.png', : [30,30], iconAnchor : [30,50] };
display360IconMarkers(map, 'moveend', pinMap, mapClicked, './images/pingImage.png', 1500);
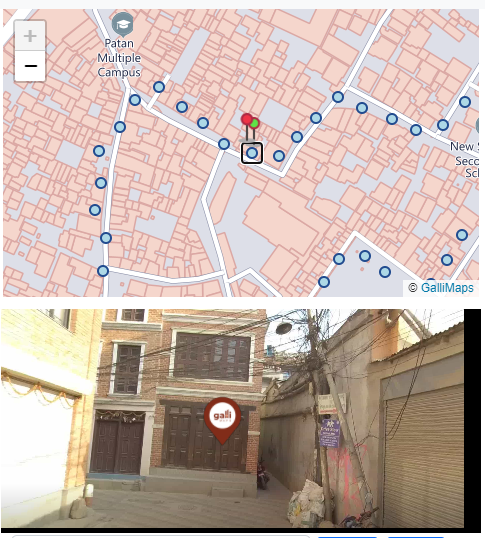
display360IconMarkers(map,mapEvents,pinMap,mapClicked,panoromaIcon,panoromaTagSize)
map | Map variable set to display 360 image markers |
mapEvents | Map bound events ("moveend","zoom") to generate 360 markers |
pinMap | Icon to show the 360 image // {iconUrl,iconSize,iconAnchor} |
mapClicked | Icon to show when clicked on map and 360 image is present // {iconUrl,iconSize,iconAnchor} |
panoromaIcon | Icon to display in 360 Panaroma Viewer |
panoromaTagSize | Icon size to display in panorama |
Note: iconUrl for each icon can be set to blank string for default icon
Autocomplete Search
This section demonstrates how to use GalliMaps async autoComplete function. This function fetches list of matched location places. JQuery has been used here for demo purpose.
SCRIPT:
autoCompleteSearch(searchText).then((result)=>{
console.log(result);
});
asyncautoCompleteSearch(searchText);
searchText | Query String |
Result Example
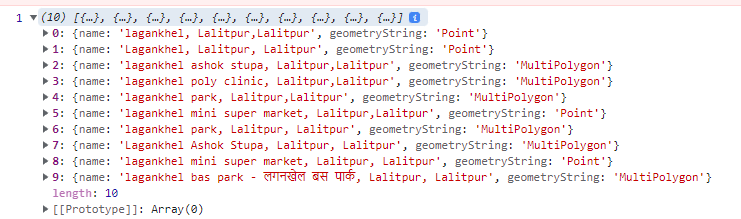
Note: Search text string length should be greater than 3.
Search
This section demonstrates how to use GalliMaps async Search function. This function fetches location data of search text provided by autoComplete function. JQuery has been used here for demo purpose.
SCRIPT:
searchData(searchText, map, iconUrl);
asyncsearch(searchData,map,iconUrl);
searchData | Query String |
map | Map variable to display searched place |
iconUrl | Icon to display searched place |
Note: After selection data will be displayed inside the map defined using <div id="map"></div>. It can be a point, line, or a polygon data.
Reverse Geocoding
This section demonstrates how to use GalliMaps async generalReverse function. A reverse geocoding search, which provides information about various locations, including house numbers, municipalities, names, places, road names, and toles.
SCRIPT:
generalReverse(27.595866,85.37997, map, true).then(response =>{ console.log(response); });
asyncgeneralReverse(lat, lng, map, customStyle, accessToken)
lat | Latitude of Location |
lng | Longitude of Location |
map | Variable mentioned above |
customStyle | This is a boolean parameter. When set to false, no data will be returned, and the information will be displayed directly on the map. When set to true, the data will be returned as shown in the image below. |
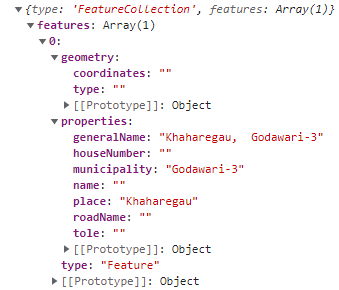
Routing
This section demonstrates how to use GalliMaps async searchRoute function to obtain routes between a source and destination location. To do so, users must provide the latitude and longitude coordinates for both locations. The output will include the distance, duration, and coordinates for the route. The request might return more than one route data as well.
SCRIPT:
var fromCoord = [27.1234,85.1234];
var toCoord = [27.3456, 85.3456];
searchRoute(fromCoord , toCoord, "driving", map, false) .then( (result) => { console.log(result); });
asyncsearchRoute(srcCoord, dstCoord, routeType, map, customStyle)
srcCoord | Source gps coordinates [latitude,longitude] |
dstCoord | Destination gps coordinates [latitude,longitude] |
routeType | Route type: driving, cycling, walking |
routetype | Type of route e.g. driving, walking, cycling |
map | Map variable |
customStyle | Boolean parameter. True = display on map, False = return as geojson for custom styling." |
Note: If the customStyle parameter is set to true, the function will return geojson data in green and red, which indicate the distance and duration taken, respectively.
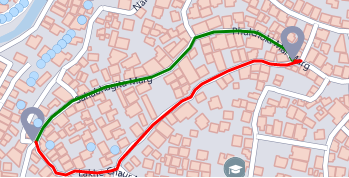
Note: If the customStyle parameter is set to false, the function will only return raw geojson data without processing and the result will not be visible in map.
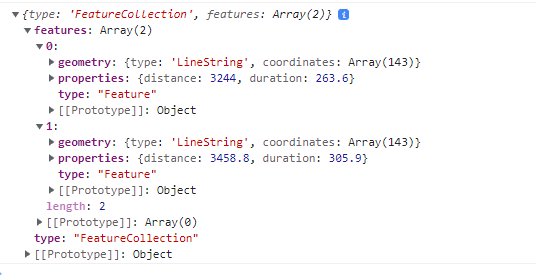