The GalliMap Vector Plugin is a JavaScript library that enables the integration of Vector GalliMaps into your web application.
Include GalliMaps JS
<script
src="https://gallimap.com/static/dist/js/gallimaps.vector.min.latest.js"></script>
DEMO WEBSITE
Display Map
This section shows how to embed GalliMaps map into website using [gallimap_plugin.js].
<!DOCTYPE html>
<html lang="en">
<head>
<title>Galli Maps</title>
<meta charset="utf-8" />
<meta http-equiv='X-UA-Compatible'
content='IE=edge'>
<meta name="viewport"
content="width=device-width,
initial-scale=1.0">
<link rel="shortcut icon"
type="image/x-icon"
href="docs/images/favicon.ico" />
</head>
<body>
<div id="map"></div>
<div id="pano"></div>
<script
src="[gallimaps.vector.min.latest.js]"></script>
<script>
// GalliMaps Options
const galliMapsObject = {
accessToken:
'ACCESS-TOKEN',
map: { container: 'map',
center: [27, 85], zoom: 20, maxZoom: 25, minZoom: 5
},
pano: { container: 'pano', },
};
// Initialize Gallimaps Object
const map = new GalliMapPlugin(galliMapsObject);
</script>
</body>
</html>
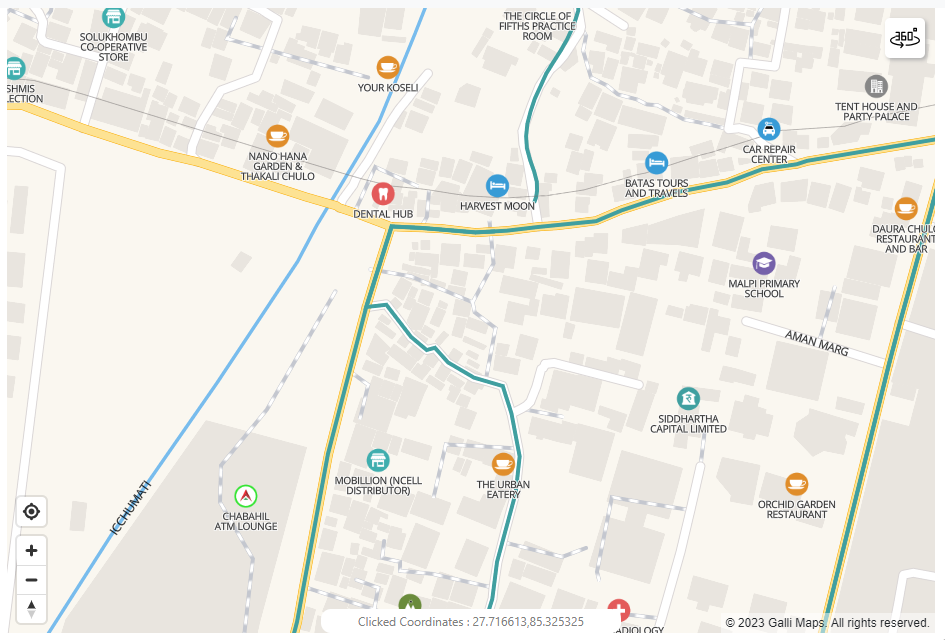
Set GalliMaps Options
const galliMapsObject = {
accessToken: 'ACCESS-TOKEN',
map: {
container: 'MAP-DIV-ID', center: [LATITUDE,
LONGITUDE], zoom: ZOOM-LEVEL, maxZoom: MAZ-ZOOM,
minZoom: MIN-ZOOM },
pano: { container:
'360-IMAGE-DIV-ID', },
};
accessToken | Required for accessing the Map and extra resource which will be provided after registering in GalliMaps |
map | Map Option Object (container, center, zoom, maxZoom, minZoom, clickable?) |
pano | 360 Image Option Object (container) |
SCRIPT:
const map = new
GalliMapPlugin(galliMapsObject);
Pin Marker
This section demonstrates how to display marker in the map and remove it
SCRIPT:
// Initialize marker object
let pinMarkerObject = {
color: "#HEXCOLOR",
draggable: BOOLEAN
latLng: [LATITUDE, LONGITUDE]
};
// Display a pin marker on the map
let
marker = map.displayPinMarker(pinMarkerObject);
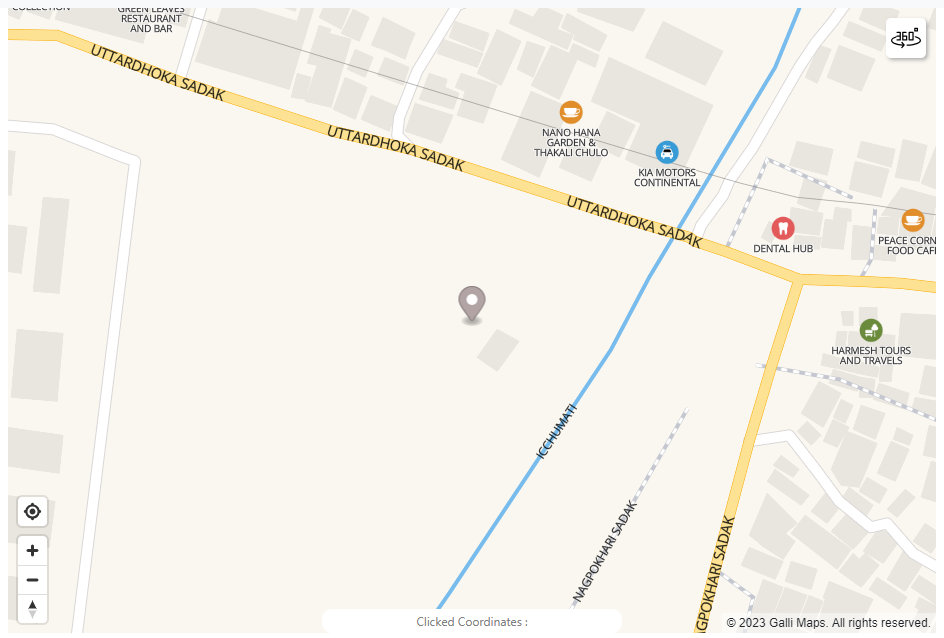
Set Pin Marker Options
color | Hex Code of marker color |
draggable | Boolean. Set marker draggable or not |
latLng | Set latitude and longitude of Marker |
// remove marker
map.removePinMarker(marker);
Autocomplete Search
This section demonstrates how to use GalliMaps async autoComplete function. This function fetches list of matched location places. JQuery has been used here for demo purpose.
SCRIPT:
map.autoCompleteSearch(searchText).then((result)=>{
console.log(result);
});
async[GALLIMAP_PLUGIN_OBJECT].autoCompleteSearch(searchText);
searchText | Query String |
Result Example
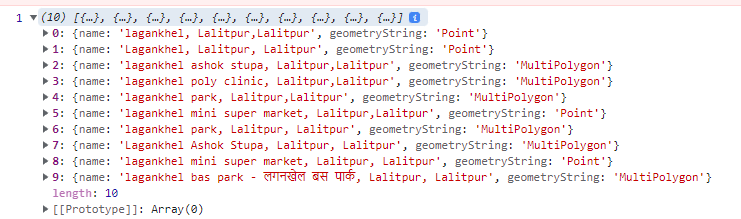
Note: Search text string length should be greater than 3.
Search
This section demonstrates how to use GalliMaps async Search function. This function fetches location data of search text provided by autoComplete function. JQuery has been used here for demo purpose.
SCRIPT:
map.searchData(searchText);
async[GALLIMAP_PLUGIN_OBJECT].search(searchData);
searchData | Query String |
Note: After selection data will be displayed inside the map defined using <div id="map"></div>. It can be a point, line, or a polygon data.
Polygon
This section demonstrates how to add and remove Custom Polygon. This function adds any polygon in geoJson Format to Map with custom shape, color and opacity.
Draw Polygon
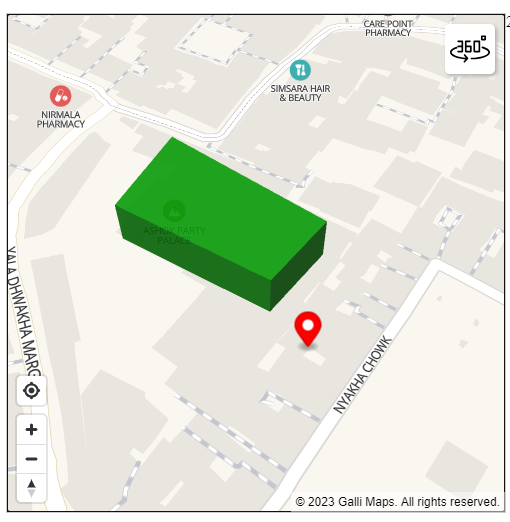
SCRIPT:
// Initialize polygonOption object
let polygonOption = {
name: "polygonname", // STRING polygon name
color: "green", // color name / HEX COLOR
opacity: 0.5 // FLOAT (0, 1)
latLng: [25.7, 85.1] // [LATITUDE, LONGITUDE]
geoJson: { // geoJson Object
"type": "Feature",
"geometry": {
"type": "Polygon",
"coordinates": [
[
[85.322097, 27.676906],
[85.322191, 27.677061],
[85.322485, 27.676872],
[85.322382, 27.676758],
[85.322097, 27.676906]
]
]
}
}
};
// Display a polygon on the map
map.drawPolygon(polygonOption);
Draw LineString
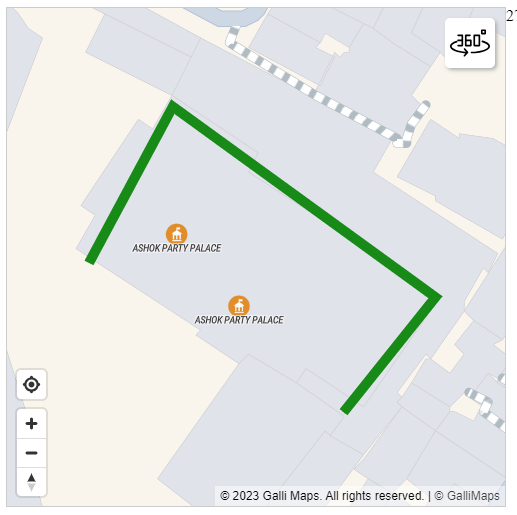
SCRIPT:
// Initialize LineString
let lineStringOption = {
name: "linestringname", // STRING polygon name
color: "green", // color name / HEX COLOR
opacity: 0.5 // FLOAT (0, 1),
width: 10 // width of the linestring,
latLng: [25.7, 85.1] // [LATITUDE, LONGITUDE]
geoJson: { // geoJson Object
"type": "Feature",
"geometry": {
"type": "LineString",
"coordinates": [
[85.322097, 27.676906],
[85.322191, 27.677061],
[85.322485, 27.676872],
[85.322382, 27.676758]
]
}
}
};
// Display a lineString on the map
map.drawPolygon(lineStringOption);
[GALLIMAP_PLUGIN_OBJECT].drawPolygon(polygonOptions);
Polygon Options
name | Polygon Name (String) |
color | Polygon Color/Hex Color (String) |
opacity | Polygon Opacity 0-1 (Float) |
height | Polygon Height (Optional in meters) |
width | PolyLine Width (Optional in meters for LineString) |
radius | Point Radius (Optional in meters for Point) |
geoJson | Polygon geoJson Object |
Remove Polygon
SCRIPT:
// Remove polygon from the map
map.removePolygon('polygonname');
[GALLIMAP_PLUGIN_OBJECT].removePolygon([POLYGON_NAME]);
Custom Click Function
This section demonstrates how to add custom click function on map. This allows user to add custom function that is triggered when clicking the map.
SCRIPT:
// GalliMaps Options
const customFunction = (event) {
// event is an object containing information about click event
console.log(event);
// get lattitude and longitude of clicked point
console.log(event.lngLat.lat); // for latitude,
console.log(event.lngLat.lng); // for longitude
..
// custom logic
}
const galliMapsObject = {
accessToken: 'ACCESS-TOKEN',
map: {
container: 'map',
center: [27, 85],
zoom: 20,
maxZoom: 25,
minZoom: 5,
clickable: true, // this needs to be true for map click event to fire
},
pano: { container: 'pano', },
customClickFunctions: [custom1], // pass multiple function when clicking the map
};
Set GalliMaps Options
const customFunction1 = (event) { // click event
object console.log(event); }
const galliMapsObject = {
accessToken:
'ACCESS-TOKEN',
map: { .. , clickable: true },
...
customClickFunctions : [customFunction1] // Array of
custom function
};
accessToken | Required for accessing the Map and extra resource which will be provided after registering in GalliMaps |
map | Map Option Object (.. , clickable = true) |
customClickFunctions | Array of custom functions |